Java基础语法(三)
文章目录
1.包机制(也就是一个文件夹)
- 为了更好地组织类,Java提供了包机制,用于区别类名得命名空间。
- 包语句得语法格式为:
pack pkg1[.pkg2[.pkg3…]];
- 一般利用公司域名倒置作为包名;例如:www.baidu.com——> com.baidu.www
- 为了能够使用某一个包得成员,我们需要在Java程序中明确导入该包。使用“import”语句可以完成此功能
import package1[.package2…].(classname|*);
2.JavaDoc的生成
-
Javadoc命令是用来生成自己API文档的
-
参数信息
(1)@author 作者名
(2)version 版本号
(3)since 指明需要最早使用的jdk版本
(4)@param参数名
(5)@return 返回值情况
(6)@throws异常抛出情况 -
Java文档的生成:
package com;/** * @author Daniel * @version 0.1 * @since 1.0 */public class Doc { String name = "Daniel"; /** * @param name * @return * @throws Exception */ public String test(String name)throws Exception{ return name; }}
打开所在目录(如下图顺序):
1.打开源码所在目录
2.输入
cmd
3.输入:
javadoc -encoding UTF-8 -charset UTF-8 Doc.java
4.回车即可生成文档
在生成文档中找到index.html双击显示如下生成文档:
思考:怎么用IDEA可以生成Javadoc
3.用户交互(键盘录入)Scanner
概述:
1、键盘录入:在程序运行的过程中,可以让用户录入一些数据,存储在内存的变量中,在后续的程序运行过程中,可以使用这些数据。
2、步骤:
第一步:导包,在类声明的上面,import java.util.Scanner;
第二步:创建键盘录入对象,Scanner sc = new Scanner(System.in);
第三步:调用sc对象的nextInt方法,获取键盘录入的整数
int x = sc.nextInt();
第四步:使用x后续处理
Scanner对象
java.util.Scanner是Java5的新特性,可以通过Scanner类来获取用户的输入。
基本语法:
Scanner s = new Scanner(System.in);
通过Scanner类的next()与nextLine()方法获取输入的字符串,在读取前我们一般使用hasNext()与hasNextLine()判断是否还有输入的数据。
next():
- 一定要读取到有效字符后才可以结束输入;
- 对输入有效字符之前遇到的空白,next()方法会自动将其去掉;
- 只有输入有效字符后才将其后面输入的空白作为分隔符或者结束符;
4. next()不能得到带有空格的字符串。
nextLine():
- 以Enter为结束符,也就是说nextLine()方法返回的是输入回车之前的所有字符。
- 可以获得空白。
public class Next_Demo { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.println("使用next方式接收:"); if(sc.hasNext()){ String st = sc.next(); System.out.println("输出的内容为:"+st); } sc.close();//凡是属于IO流的类如果不关闭会一直占用资源,要养成好习惯用完就关掉 }}
运行结果为:
使用next方式接收:
Hello World
输出的内容为:Hello
Process finished with exit code 0
public class NextLine_Demo { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("使用nextLine方法接收:"); if(scanner.hasNextLine()){ String str = scanner.nextLine(); System.out.println("输出的内容为:"+str); } scanner.close(); }}
运行结果为:
使用nextLine方法接收:
Hello World
输出的内容为:Hello World
Process finished with exit code 0
public class JianPanLuRu { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("请输入:"); String str1 = scanner.next(); System.out.println("str1:"+str1); String str2 = scanner.nextLine(); System.out.println("str2:"+str2); scanner.close(); }}
运行结果为:
请输入:
Hello World
str1:Hello
str2: World
Process finished with exit code 0
4.顺序结构
- Java的基本结构就是顺序结构,除非特别指明,否则就按照顺序一句一句执行
- 顺序结构是最简单的算法结构。代码从上到下,从左到右依次执行
语句与语句之间,框与框之间是按从上到下的顺序进行的,它是由若干个依次执行的步骤组成的,它是任何一个算法都离不开的一种基本算法结构。
public class ShunXuDemo { public static void main(String[] args) { System.out.println("demo1"); System.out.println("demo2"); System.out.println("demo3"); System.out.println("demo4"); System.out.println("demo5"); }}
运行结果为:
demo1
demo2
demo3
demo4
demo5
Process finished with exit code 0
5.选择结构(分支结构)
1. if单选择结构
很多时候需要去判断一个东西是否可行,这样一个过程在程序中用if语句来表示
语法
if(布尔表达式){
//如果布尔表达式为true将执行的语句
}
public class IfDemo { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("Please input into:"); String s = scanner.nextLine(); if(s.equals("Hello")){//equals比较字符串的相等问题 System.out.println(s); } System.out.println("End"); scanner.close(); }}
运行结果为:
Please input into:
Hello
Hello
End
Process finished with exit code 0
2. if双选择结构
语法:
if(布尔表达式){
//如果布尔表达式的值为true
}else{
//如果布尔表达式的值为false
}
public class IfShuanXuanZe { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("Please input into score:"); int score = scanner.nextInt(); if(score >= 60){ System.out.println("及格"); } if(score < 60){ System.out.println("不及格"); }else{ System.out.println("不合格"); }}
运行结果:
Please input into score:
59
不及格
Process finished with exit code 0
3. if多选择结构
public class IfDuoXuanZe { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); /** * if语句至多有1个else语句,else语句在所有的else if语句之后。 * if 语句可以有若干个else if 语句,他们必须在else语句之前。 * 一旦其中一个else if语句检测为true,其它的else if以及else语句都将跳过执行。 */ System.out.println("请输入成绩:"); int score = scanner.nextInt(); if(score ==100){ System.out.println("恭喜满分"); }else if(score >= 90 && score <100){ System.out.println("A级"); }else if(score >= 80 && score < 90){ System.out.println("B级"); }else if(score >= 70 && score <80){ System.out.println("C级"); }else if (score >= 60 && score < 70){ System.out.println("D级"); } else { if(score >= 0 && score < 60){ System.out.println("不及格"); }else{ System.out.println("你输入的成绩不合格"); } } scanner.close(); }}
运行结果:
请输入成绩:
100
恭喜满分
Process finished with exit code 0
4. 嵌套的if结构
(1)使用嵌套的if…else语句是合法的。也就是说你可以在另一个if或者else if语句中使用if 或者else if语句。你可以像if语句一样嵌套else if…else. 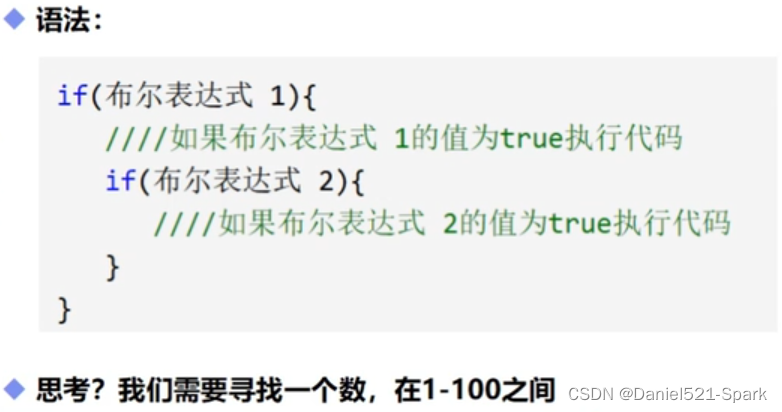
- switch多选择结构
(1)switch:表示英文中的开关,每个条件都像一扇门,开着的,就可以执行,否则就到下一扇门判断。
(3)执行流程:
1、计算表达式的值,分别和各个常量匹配
2、如果和常量1匹配,那么就执行语句体1,break,switch语句就结束了
3、如果和常量1不匹配,那么就判断是否和常量2匹配,如果匹配就执行语句体2,break,switch语句就结束了
4、以此类推,如果常量1~常量n全都没有和表达式的值匹配,那么就执行default中的内容,switch语句结束。
public class Switch_Daniel { public static void main(String[] args) { String c = "Daniel"; switch (c){ case "Daniel": System.out.println("Daniel"); break; case "Wendy": System.out.println("Wendy"); break; case "Kendra": System.out.println("Kendra"); break; case "Guo": System.out.println("Guo"); break; default: System.out.println("没有找到哦"); /*** 1、单词解释:* switch:开关;case:情况;break:破坏、退出;default:默认* 2、表达式:* Jdk1.5之前:byte、short、char、int* Jdk1.5:枚举类型* Jdk7:String* 3、case后面只能跟常量,不能跟变量* 4、每个case的语句体后面,一定要跟上一个break语句,否则就无法结束switch语句,并且发生case穿透。Case穿透的利用:多个情况如果是相同的执行逻辑,那么就可以使用case穿透。* 5、Default语句:可以写也可以不写。如果不写,就有可能一个语句都执行不到。建议把default语句加上。* 6、Default语句可以写在任意位置,无论写在什么位置,都是最后去匹配,建议把default语句写在最后。* 7、Switch语句结束的条件:碰到了break语句;碰到了右大括号*/ } }}
运行结果:
Daniel
Process finished with exit code 0
反编译(拓展)
java-----class(字节码文件)-----反编译(IDEA)
If语句和switch语句的区别
1、相同点:if语句和switch语句都可以表达分支结构
2、不同点:
(1)if语句格式相对灵活,更加强大,switch语句相对弱小。所有的switch语句都可以用if语句完成
(2)if语句更多的是做一些连续性的判断,switch语句更多做的是离散型一个匹配
(3)if语句每次都需要获取表达式的值,而switch只获取一次表达式的值,所以相对switch的效率高一些。
6.循环结构
1、对于某些需要重复执行的,相同或者相似的语句,使用某种格式来完成对代码的简化。
2、实现的语句:
while语句【常用】do...while语句for语句【常用】在Java5中引入了一种主要用于数组的增强型for循环
while语句
public class While_Daniel { public static void main(String[] args) { //输出1-50 int i = 0; while(i < 50){ i++; System.out.println(i); } }}
运行结果:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
Process finished with exit code 0
public class While_SiKao { public static void main(String[] args) { //思考:计算1+2+3+…+100=? int i = 0; int sum = 0; while(i <= 100){ sum = sum +i; i++; } System.out.println(sum); }}
运行结果为:
do…while语句
public class DoWhile_Daniel { public static void main(String[] args) { int i = 0; int sum = 0; do{ sum+=i; i++; }while(i <= 100); System.out.println(sum); }}
运行结果:
5050
Process finished with exit code 0
while和do…while的区别
public class While_DoWhile_Daniel { public static void main(String[] args) { int a = 0; while(a < 0){ System.out.println(a); } System.out.println("------------------"); do{ a++; }while (a < 0); System.out.println(a); }}
运行结果为:
------------------
1
Process finished with exit code 0
For循环
1、格式
for (初始化语句 ; 循环的条件表达式 ; 初始化变量的自增 ) {
循环体语句;
}
2、执行流程:
1、执行初始化语句
2、计算循环的条件表达式
3、如果计算为false,就结束for循环
4、如果计算为true,就执行循环体语句
5、执行初始化变量的自增
6、回到第2步
3、说明:
1、初始化语句,声明一个变量,用于记录循环次数
2、循环的条件表达式,控制循环的条件
3、初始化变量的自增,将初始化语句中声明的变量,进行变化,一般是向着循环不能执行的方向变化
4、循环体语句,就是那个要重复执行的内容
IDEA中输入技巧 100.for回车 相当于for(int i=0;i<100;i++){}
(1)
public class While_For_Daniel { public static void main(String[] args) { int a = 1; while (a <= 20){ System.out.println("a="+a); a++; } System.out.println("while循环结束"); for (int i = 1; i <= 20; i++) { System.out.println("i="+i); } System.out.println("for循环结束"); }}
运行结果为:
a=1
a=2
a=3
a=4
a=5
a=6
a=7
a=8
a=9
a=10
a=11
a=12
a=13
a=14
a=15
a=16
a=17
a=18
a=19
a=20
while循环结束
i=1
i=2
i=3
i=4
i=5
i=6
i=7
i=8
i=9
i=10
i=11
i=12
i=13
i=14
i=15
i=16
i=17
i=18
i=19
i=20
for循环结束
Process finished with exit code 0
练习1.
public class For_JiOu_Daniel { public static void main(String[] args) { int oddSum = 0; int eventSum = 0; for (int i = 0; i < 100; i++) { if(i % 2 == 0){ oddSum+=i; }else{ eventSum+=i; } } System.out.println("1到100偶数之和为"+oddSum); System.out.println("1到100奇数之和为"+eventSum); }}
运行结果为:
练习2.
public class For_ZhenChu_Daniel { public static void main(String[] args) { for (int i = 0; i < 1000; i++) { if(i % 5== 0){ System.out.print(i+"\t"); } if(i%(5*3) == 0){ System.out.println(); // System.out.println("\n"); } //print 输出不换行 //println 输出换行 } }}
运行结果为:
0
5 10 15
20 25 30
35 40 45
50 55 60
65 70 75
80 85 90
95 100 105
110 115 120
125 130 135
140 145 150
155 160 165
170 175 180
185 190 195
200 205 210
215 220 225
230 235 240
245 250 255
260 265 270
275 280 285
290 295 300
305 310 315
320 325 330
335 340 345
350 355 360
365 370 375
380 385 390
395 400 405
410 415 420
425 430 435
440 445 450
455 460 465
470 475 480
485 490 495
500 505 510
515 520 525
530 535 540
545 550 555
560 565 570
575 580 585
590 595 600
605 610 615
620 625 630
635 640 645
650 655 660
665 670 675
680 685 690
695 700 705
710 715 720
725 730 735
740 745 750
755 760 765
770 775 780
785 790 795
800 805 810
815 820 825
830 835 840
845 850 855
860 865 870
875 880 885
890 895 900
905 910 915
920 925 930
935 940 945
950 955 960
965 970 975
980 985 990
995
Process finished with exit code 0
练习3.
public class JiuJiuChengFaBiao_Daniel {/** * 1.先打印第一列 * 2.然后再把固定的1再用一个循环包起来 * 3.去掉重复项,j <= i * 4.调整样式 */ public static void main(String[] args) { for (int i = 1; i <= 9; i++) { for (int j = 1; j <= i; j++) { System.out.print(i+"*"+j+"="+(i*j)+"\t"); } System.out.println(); } }}
运行结果为:
for语句格式的注意事项
1、初始化语句,声明一个变量,用于记录循环次数,只执行一次。
2、条件表达式,必须是boolean类型的运算结果
3、循环体语句,在初始化变量自增之前,执行的
4、初始化变量的自增,可以是自增、也可以是自减,也可以一次自增多步
左大括号之前,不要有分号,否则for循环无法控制大括号中的语句。
增强for循环(先了解后面会详细记录)
public class ForEach_Daniel { public static void main(String[] args) { int [] numbers = {10,20,30,40,50}; for (int i = 0; i < 5; i++) { System.out.println(numbers[i]); } System.out.println("---------------"); for (int x: numbers) { System.out.println(x); } }}
运行结果为:
三种循环语句的区别
1、dowhile语句和其他两种语句的区别:
dowhile语句至少可以执行一次,另外两种有可能一次都执行不了
2、while语句和for的区别:
1、代码层面:while语句声明的初始化变量,在while结束之后,还能继续使用;for中声明的初始化变量,在for结束之后,就无法使用了。
2、设计层面:while语句一般用于描述相对模糊的范围,其他的条件;for用于描述相对准确,知道循环次数的循环
7. break和Continue
1、跳转语句:在循环的循环体语句中,结束循环,控制循环,使用的语句。
2、continue语句:
结束本次循环,继续下次循环
3、break语句:
结束当前层次的循环
4、return语句:
结束当前的方法
5、System.exit(0);
用于结束虚拟机
打印三角形:
public class DaYinSanJiaoXing_Daniel { public static void main(String[] args) { for (int i = 1; i < 5; i++) { for (int j = 1;j <= i;j++){ System.out.print("*"); } System.out.println(); } }}
结果显示为: